IDLE#
Video Tutorial
Tip
You can copy and paste code in the gray code blocks by hovering your mouse over the block and pressing the icon appearing in the top right.
IDLE is an integrated development environment (IDE) installed automatically with Python. It provides you with an environment where you can write Python scripts and run them or write Python code line by line to execute immediately.
IDLE is lightweight, simple, and great for introducing how to do coding projects. Therefore, it is well suited for beginners.
Warning
We only recommend introductory students to use IDLE while following 02002/02003.
There are other more advanced IDE’s that has much greater functionality than IDLE.
Getting started#
Open IDLE .
Open up a terminal (see here) and type, then press Enter
idle
You will see the following screen:

The text you see indicates that you have opened a Python Shell. The lines starting with “>>>” are meant for Python code. Try typing or pasting in the following and press enter.
print("hello")
Open up a terminal (see here) and type, then press Enter
idle3
You will see the following screen:
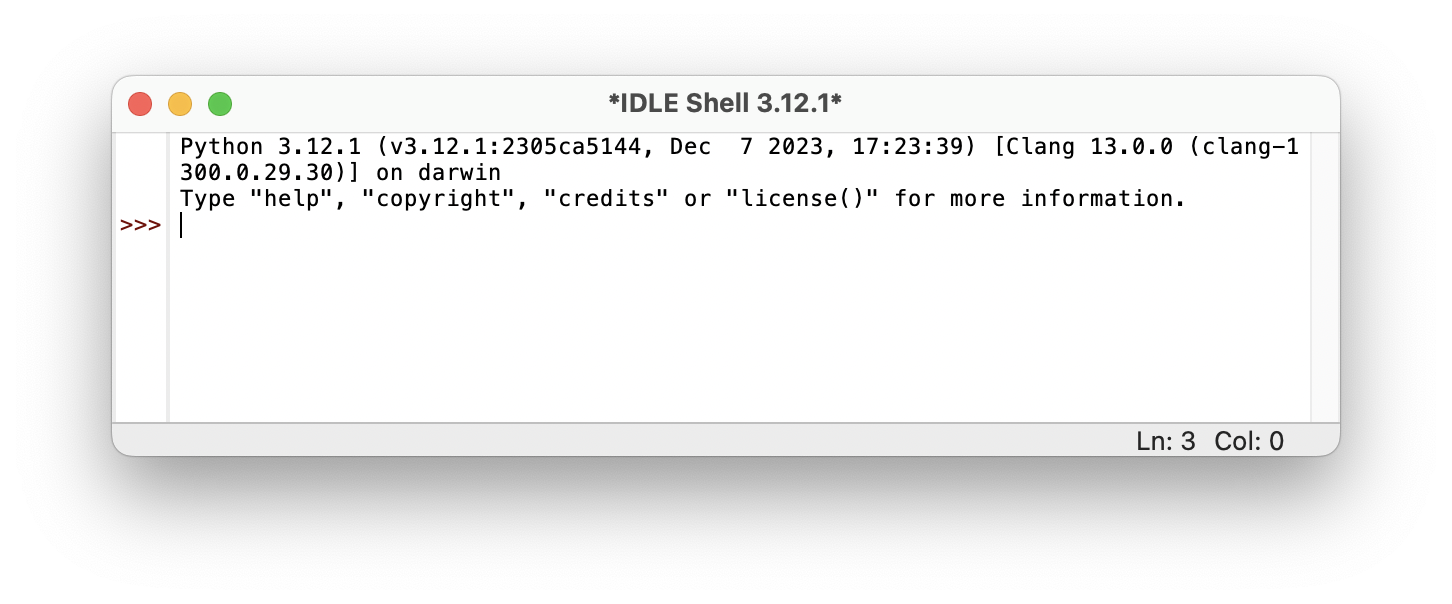
The text you see indicates that you have opened a Python Shell. The lines starting with “>>>” are meant for Python code. Try typing or pasting in the following and press enter.
print("hello")
Creating and running scripts#
A Python script is a file with Python code. Once the script is run, all the code is executed. This is great for larger jobs and allows for reuse of your code.
Go to
in the IDLE menu to create a Python script.You should see a blank page. Type or paste the following code
# This program is for adding 2 numbers # Declaring the variables num1 = 18 num2 = 29 # Doing the addition sum = num1 + num2 # Printing the sum print(f"Sum of {num1} and {num2} equals {sum}")
Go to
and save the script assum.py
Run the script. In the top menu, go to
(or press F5). You should now see some output.
Go to
in the IDLE menu to create a Python script.You should see a blank page. Type or paste the following code
# This program is for adding 2 numbers # Declaring the variables num1 = 18 num2 = 29 # Doing the addition sum = num1 + num2 # Printing the sum print(f"Sum of {num1} and {num2} equals {sum}")
Go to
and save the script assum.py
Run the script. In the top menu, go to
(or press F5). You should now see some output.
You can now modify and run the script as much as you want.
As mentioned, the IDLE is best suited for simple projects. Later during the 1st semester you will be introduced to Visual Studio Code.